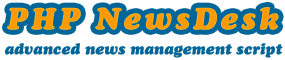
Coding Standards
These are the coding standards for PHP NewsDesk, and all attempts should be made to follow these standards as closely as possible.
Formatting and Layout
Indentation
Code should be indented wherever necessary (e.g. within control structures such as loops and conditionals). This helps to organise code and group code blocks. Indentation should be applied consistently throughout your code.
Tabs (i.e. hard tabs) should be used as the indentation mechanism in favour of spaces. Tabs are preferred because there is no fixed indent width; this allows each developer to specify how wide a tab should appear in their code editor settings, without it breaking the layout of the actual files.
There should also be an initial indent between the opening and closing PHP tags (i.e. <?php and ?>) to signify that the code is within a PHP block.
For example, this is wrong:
<?php echo 'Hello World'; ?>
This is right:
<?php echo 'Hello World'; ?>
Line Endings
Because many web servers run on UNIX-based platforms, UNIX-style line endings (that is, a line feed character only) are preferred to Windows-style line endings (carriage return and line feed characters) and Mac-style line endings (a carriage return character only).
Additionally, ensure that there are no trailing new lines at the end of your files. Any whitespace at the end of a file will be sent as output to the browser and may cause problems if HTTP headers are to be sent by another file.
Line Length
Because most decent code editors support automatic word wrapping, in that long lines are wrapped to avoid horizontal scrolling, no limits on line length are enforced. However, if a line is so long that it is unreadable, divide it into several lines.
Code Layout
Braces
Braces should always be used in control structures, even if they are not necessary, such as with single-line conditionals. Braces help to visually group code blocks, adding clarity to the code and reducing mistakes.
For example, while the following code is perfectly valid, it is wrong:
if ($posts == 1) do_something();
Instead, the code should be formatted like this:
if ($posts == 1) { do_something(); }
Braces should be positioned in your code using the K&R style, where the opening brace is placed on the same line as the keyword.
For example, this is wrong:
if ($posts == 1) { do_something(); }
And so is this:
if ($posts == 1) { do_something(); }
While this is right:
if ($posts == 1) { do_something(); }
Spaces
There should always be spaces between tokens. By this I mean write code as you would write in English (or whatever your native language may be). Just as you would put spaces between words, put spaces between variables and operators.
However, just like with English, spaces should not go before commas or semi-colons, or after an opening bracket or before a closing bracket.
The following examples demonstrate the wrong way followed by the right way:
$posts=1; $posts = 1;
if($posts==1) if ($posts == 1)
while ( $posts<2 ) while ($posts < 2)
do_something( 1 , 2 , 3 ); do_something(1, 2, 3);
Brackets
To avoid confusion with operator precedence in conditional statements, brackets should surround each condition. This makes it clear what is being compared without having to remember the rules of operator precedence.
For example, the following conditional is wrong and confusing:
if ($date == 29 && $month == 2 || $is_leap_year)
This is a lot more understandable and leaves no room for confusion:
if ((($date == 29) && ($month == 2)) || ($is_leap_year))
Strings
Strings should be quoted using single quotes. Not only is this faster for the PHP parser to process, it won't lead to unexpected results when using symbols such as dollar signs in a string.
For example, this is wrong:
echo "Hello World";
This is right:
echo 'Hello World';
If you need to include variables, constants, or return values from functions or methods as part of a string, use concatenation. In other words, join the strings or symbols that represent strings with a period symbol.
For example:
$welcome_message = 'Good morning, '.$user_name.'!';
There should not be any spaces between the period symbols in order to keep the string more concise and like the final concatenated result as possible.
Associative Array Keys
When using strings as keys in associative arrays, always quote the key value using single quotes.
For example, this is wrong:
$posts = $user_info[posts];
And this is right:
$posts = $user_info['posts'];
Naming Symbols
All symbols for all languages, be it variable names in PHP or function names in JavaScript, should always be descriptive, but concise.
Variables
PHP
Variables names in PHP should all be in lowercase, and underscores should be used to separate words.
For example, this variable name is right:
$num_visits
These variable names are wrong:
$numvisits $numVisits $NumVisits
JavaScript
Variable names in JavaScript should have the first letter of all words in uppercase.
For example, this variable name is right:
NumVisits
These variable names are wrong:
numvisits numVisits num_visits
Constants
Constant names should always be in all uppercase, and underscores should be used to separate words.
For example, this constant name is right:
MAX_POSTS
These constant names are wrong:
maxposts MaxPosts Max_Posts
Functions
PHP
Function names in PHP should be in all lowercase, and underscores should be used to separate words.
For example, this function name is right:
do_something();
These function names are wrong:
dosomething(); doSomething(); DoSomething();
JavaScript
Function names in JavaScript should be in studly caps/camel caps, where the first letter of the first word is in lowercase, and first letter of all subsequent words are in uppercase.
For example, this function name is right:
doSomething();
These function names are wrong:
dosomething(); DoSomething(); do_something();
Classes
Class names in both PHP and JavaScript should have the first letter of all words in uppercase.
For example, this class name is right:
MyDatabase
These class names are wrong:
mydatabase myDatabase my_database
Methods
Method names in both PHP and JavaScript should be in studly caps/camel caps, where the first letter of the first word is in lowercase, and first letter of all subsequent words are in uppercase.
For example, this method name is right:
exportPost();
These method names are wrong:
exportpost(); ExportPost(); export_post();
Documentation
Comments
Use comments whenever it is unclear as to what purpose a line or lines of code serves. Comments should explain the logic flow, and should always be used to clarify code.
For single-line comments, use C++ style comments (i.e. //) rather than Shell/Perl style comments (i.e. #). If a comment spreads across multiple lines, use C-style comments (i.e. /* ... */).
API Documentation
Each file, constant, include, class, property, method, and function should be accompanied by a phpDocumentor comment block explaining its use and its syntax. This helps people to use the code without having to read through the code itself.
Things to Avoid
Avoid the following at all times:
- Global variables should not be used. They cause too many problems and potential conflicts with other code, making them a nightmare to debug. If you need to access something in other parts of your script, use alternatives instead, such as singleton classes.
- Short open tags (i.e. <?) should not be used, as they are not enabled by all web hosting providers and conflict with XML tags. Similarly do not use ASP-style tags (i.e. <% and %>) either. Always use the long PHP open tags (i.e. <?php).
- Try to avoid deeply nested code wherever possible by using the break or continue keywords in loops, or by returning values or throwing exceptions at the beginning of functions/methods instead of wrapping them in large or multiple if statements.
- The echo or print PHP functions should not be used to send output to the browser. Instead, templates should be used to separate the page output from the business logic. The echo or print functions may be used for debugging purposes only.